Masking Third Party Dependencies
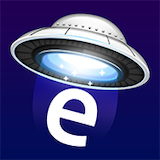
This post is brought to you by Emerge Tools, the best way to build on mobile.
Since shipping Elite Hoops, I’ve found myself using a few third-party dependencies. Namely, Mixpanel for some very lightweight analytics. Did the person open this tab, see that thing, etc.
Today’s little post is simply a reminder to abstract this code away. For example, instead of this:
func didTapNewTeam() {
Mixpanel.mainInstance().track(event: "Created a New Team")
// Code to create a new team
}
Opt for a light Struct to house that logic:
struct Telemetry {
static func sendDidTapNewTeam() {
guard !UIDevice.current.isSimulator else { return }
Mixpanel.mainInstance().track(event: "Created a New Team")
}
}
Now, your call site is none-the-wiser:
func didTapNewTeam() {
Telemetry.sendDidTapNewTeam()
// Code to create a new team
}
However, this can save you some heartache in the end. To wit - when I began Elite Hoops, I was using Telemetry Deck. While it’s a nice product, it didn’t quite click for me. But, since I used this pattern all over already, changing over to Mixpanel was a one line change. I only had to edit didTapNewTeam()
. If I hadn’t, I’d be making several updates throughout the app where I used any analytics.
In college, I remember seeing this pattern floated around as the “repository pattern” - wherein your “DAO” (data access object - we’re going real .NET here for a minute) had no clue about the inner-working of your actual CRUD code. It just called save()
, delete()
and update()
. But, whichever database service or API you were using was all housed within those functions.
Whatever you call it, be it abstraction or some sort of pattern, I recommend masking any third-party code behind your own object or struct. By nature, you’re giving up some control when you use it, and for that reason third-party code tends to be inherently obstreperous at some point. If you mask it away, though, it makes refactoring affairs a breeze.
Until next time ✌️.