Showing What's New Screens using @AppStorage
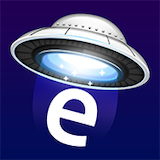
This post is brought to you by Emerge Tools, the best way to build on mobile.
The “What’s New” or “Release Notes” view are found in iOS apps the world over. Elite Hoops is no exception:
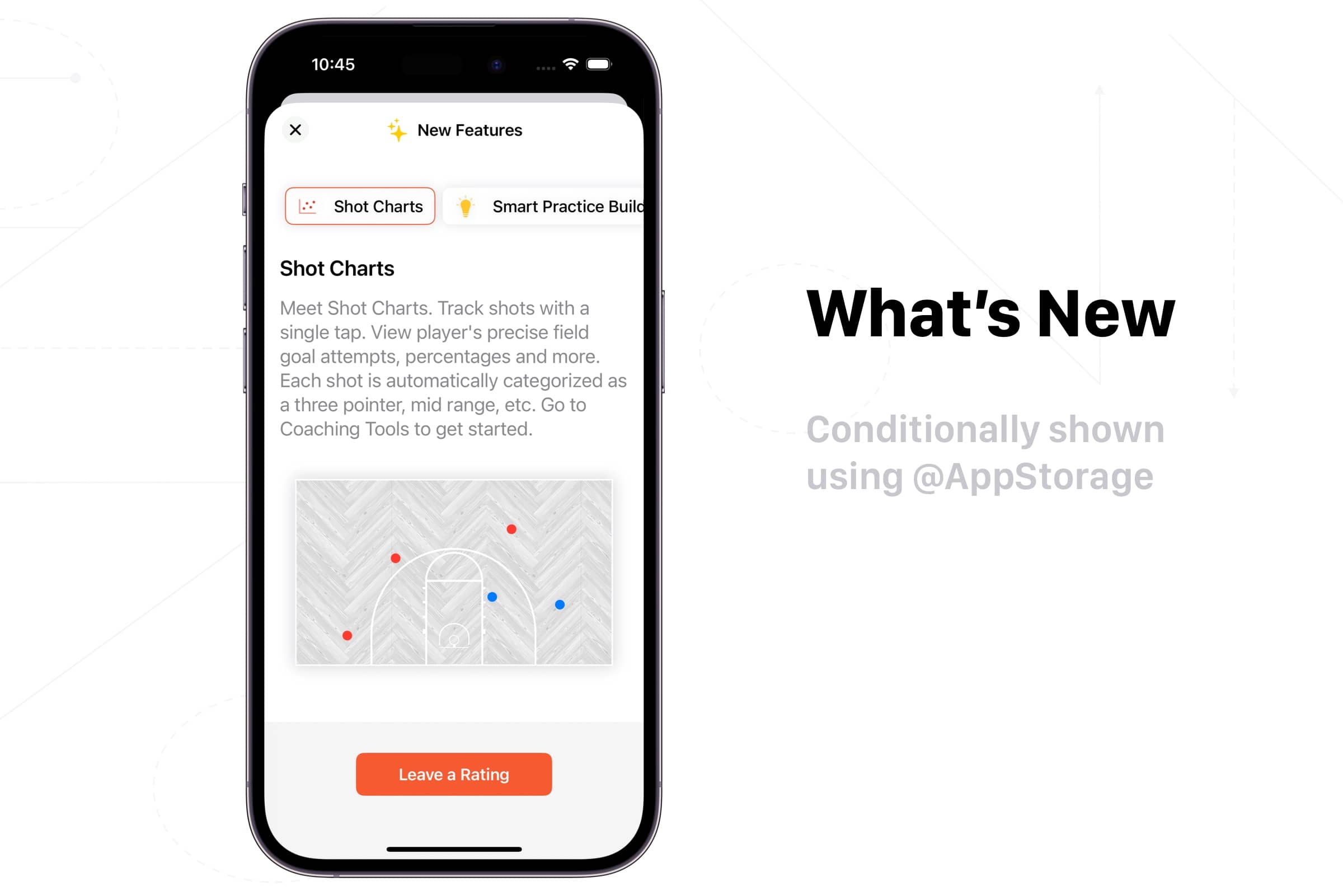
With SwiftUI and @AppStorage
, this type of thing requires me to change, quite literally, one character in my codebase when I want to fire it:
private struct AppSettingsKey: EnvironmentKey {
static var defaultValue: AppSettings { .init() }
}
extension EnvironmentValues {
var appSettings: AppSettings {
get { self[AppSettingsKey.self] }
set { self[AppSettingsKey.self] = newValue }
}
}
// MARK: App Settings
final class AppSettings: ObservableObject {
@AppStorage("hasSeenWhatsNewV12") var hasSeenWhatsNew: Bool = false
}
I version my what’s new stuff, so when there is something to show, I simply increment the number:
@AppStorage("hasSeenWhatsNewV12") var hasSeenWhatsNew: Bool = false
// Becomes...
@AppStorage("hasSeenWhatsNewV13") var hasSeenWhatsNew: Bool = false
Since the app storage macro uses UserDefaults
under the hood, it’ll recognize this as a new key — which defaults to false
:
@main
struct EliteHoopsApp: App {
private let deepLinker: DeepLinker = .init()
var body: some Scene {
WindowGroup {
TheViews()
.sheet(item: $deepLinker.sheetLinkedView) { deepLink in
switch deepLink {
case .whatsNew:
WhatsNewView()
default:
EmptyView()
}
}
.environment(\.appSettings, appSettings)
.environment(deepLinker)
.task {
showPostLaunchAnnouncementsIfNeeded()
}
}
}
private func showPostLaunchAnnouncementsIfNeeded() {
guard deepLinker.linkedView == nil else { return }
if !appSettings.needsViewOnboarding &&
!appSettings.hasSeenWhatsNew &&
appSettings.numberOfOpens >= 2 {
appSettings.hasSeenWhatsNew = true
deepLinker.sheetLinkedView = .whatsNew
}
}
}
And that’s it — once the user has:
- Gone through onboarding.
- Opened the app more than two times.
- And has not seen the latest what’s new view, it’ll show.
A lot of developers will point out that nobody really looks at these things. Maybe they’re right, but I love working on it. It’s a dopamine hit that tells me I’ve finished up a big feature — and I tell people about it succintly and interactively.
And there’s no fluff here. Elocution is for the stage. In apps? A well-timed “What’s New” screen does all the talking you need — and SwiftUI can do it one character.
Until next time ✌️